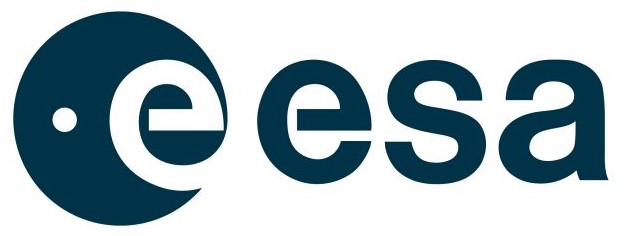
European Space Agency¶
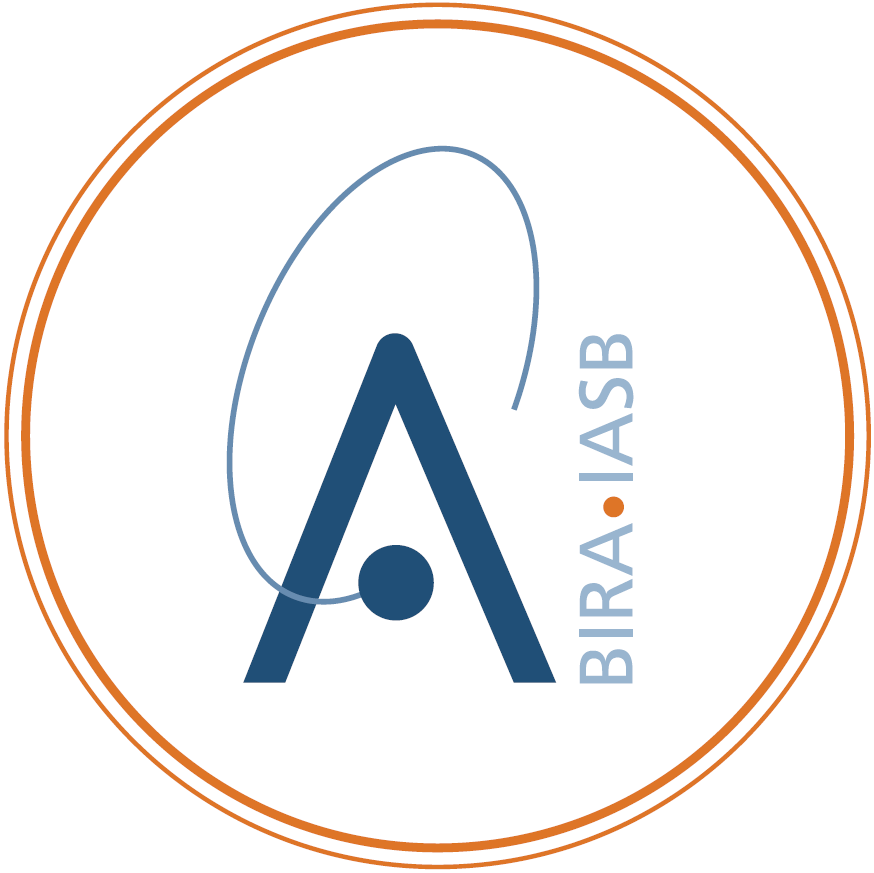
Royal Belgian Institute for Aeronomy¶

Ex. #5. namelist to initialize UNILIB¶
Description¶
This example illustrates the use of a namelist to input data to initialize UNILIB. Namelists were often implemented in FORTRAN 77 and have been a feature of FORTRAN since FORTRAN 90. A namelist specifies a list of variables whose values may be easily read or written in an ASCII file. Namelist input takes the form:
&groupName
variable = value
[,variable = value ,...]
/
where
groupName
is the name of the namelist that contains the different variables. The namelist is defined in the Fortran program with the statementNAMELIST
.In this example, a namelist is defined to initialize the UNILIB library . The namelist includes all the data useful to call the subroutines
um510()
,um520()
andua610()
. A specific feature of the Fortran namelists allows to define default values for the different variables: when a variable is omitted in the namelist file, its value is not modified by theREAD
statement. The initialisation of UNILIB is realised by the subroutineinitul()
. The argumentkunit
corresponds to the unit number of the namelist file andklist
to a switch to control the printing of the namelist contents. The namelist is calledunilib
and all its variable are listed in the table below. Note that other variables, such as the solar wind pressure, can be added in the namelist.
Variable |
Default |
Description |
---|---|---|
kinner |
0 |
Internal magnetic field model number |
bltime |
1995.0 |
Epoch for internal magnetic field model |
kouter |
0 |
External magnetic field model number |
datemagn |
1995, 1, 1, 0, 0, 0 |
Date and time for magnetic evaluation (Y,M,D,h,m,s) |
val_kp |
0.0 |
Geomagnetic activity index Kp |
dst |
-30.0 |
Geomagnetic activity index Dst |
winddens |
25.0 |
Density of solar wind |
windvel |
300.0 |
Velocity of solar wind |
kmflg |
0 |
Control of the magnetic moment |
katmo |
1 |
Atmospheric model number |
dateatmo |
1995, 1, 1, 0, 0, 0 |
Date and time for atmospheric evaluation (Y,M,D,h,m,s) |
rzss |
80.0 |
Sun spots number |
f107, f107a |
70.0, 50.0 |
Solar radio flux, dayly and averaged |
max_kp |
3.0 |
Maximum value of Kp |
ap() |
20.0, 20.0, 20.0, 20.0, 20.0, 20.0 |
Activity index Ap |
kflag |
see UC150 |
Internal switches for the atmospheric models (MSIS, IRI, …) |
Source¶
PROGRAM example5
C
INTEGER*4 kunit
LOGICAL*4 list
C
C open the namelist file ex5.nml
C
kunit = 1
OPEN( UNIT=kunit, FILE='ex5.nml', STATUS='old')
C
C initialize the library
C
list = .TRUE.
CALL initul (kunit, list)
C
C close the namelist file
C
CLOSE (kunit)
C
END
C -----------------------------------------------------------------
SUBROUTINE initul (kunit, list)
C
C initialisation of UNILIB with a namelist
C
C kunit : unit number of the namelist file
C list : when set to true, prints the namelist contents
C
INCLUDE 'unilib.h'
C
INTEGER*4 kunit
LOGICAL*4 list
C
C common blocks UC150 and UC190
C
COMMON /UC150/matm, ntspec, nnspec, kspec, kflag
C
TYPE(zatm) matm
INTEGER*4 ntspec, nnspec, kspec(30)
INTEGER*4 kflag(50)
C
COMMON /UC190/ prop, stepx, stpmin, umsq, upsq, uk2, uk3,
: epskm, epsrel, stplst, xclat, kmflg, nxstp
C
REAL*8 prop, stepx, stpmin
REAL*8 umsq, upsq, uk2, uk3
REAL*8 epskm, epsrel, stplst, xclat
INTEGER*4 kmflg, nxstp
C
C definition of the namelist 'unilib'
C
C note that datemagn and dateatmo are integer and
C max_kp is a float
C
INTEGER*4 kinner, kouter, datemagn(6), katmo, dateatmo(6)
REAL*8 bltime, dst, val_kp, winddens, windvel
REAL*8 rzss, f107, f107a, max_kp, ap(6)
C
NAMELIST /unilib/ kinner, bltime, kouter, datemagn, val_kp, dst,
: winddens, windvel, kmflg, katmo, dateatmo,
: rzss, f107, f107a, max_kp, ap, kflag
C
C other variables
C
INTEGER*4 kout, kini, ifail
CHARACTER*32 label
TYPE(zdat) mdm, mda
REAL*8 param(10)
C
C initialize UNILIB
C
kout = 6
kini = 1
C
CALL UT990 (kout, kini, ifail)
IF( ifail .LT. 0 )THEN
WRITE(kout,1000) 'UT990',ifail
STOP
ENDIF
C
C set the default values
C
C note that kmflg and kflag values are set by
C the subroutine UT990
C
kinner = 0
bltime = 1995.0
kouter = 0
datemagn(1) = 1995
datemagn(2) = 1
datemagn(3) = 1
datemagn(4) = 0
datemagn(5) = 0
datemagn(6) = 0
val_kp = 0.0
dst = -30.0
winddens = 25.0
windvel = 300.0
katmo = 1
dateatmo(1) = 1995
dateatmo(2) = 1
dateatmo(3) = 1
dateatmo(4) = 0
dateatmo(5) = 0
dateatmo(6) = 0
rzss = 80.0
f107 = 70.0
f107a = 50.0
max_kp = 3.0
ap(1) = 20.0
ap(2) = 20.0
ap(3) = 20.0
ap(4) = 20.0
ap(5) = 20.0
ap(6) = 20.0
C
C read the namelist
C
READ(kunit,unilib,IOSTAT=ifail)
IF( ifail.ne.0 )THEN
WRITE(kout,1010) ifail
STOP
ENDIF
C
C transform the date
C
mdm%iyear = datemagn(1)
mdm%imonth = datemagn(2)
mdm%iday = datemagn(3)
mdm%ihour = datemagn(4)
mdm%imin = datemagn(5)
mdm%secs = datemagn(6)
CALL UT540 (mdm)
C
mda%iyear = dateatmo(1)
mda%imonth = dateatmo(2)
mda%iday = dateatmo(3)
mda%ihour = dateatmo(4)
mda%imin = dateatmo(5)
mda%secs = dateatmo(6)
CALL UT540 (mda)
C
C set the different models
C
CALL UM510 (kinner, bltime, label, kout, ifail)
IF( ifail .LT. 0 )THEN
WRITE(kout,1000) 'UM510',ifail
STOP
ENDIF
C
param(1) = val_kp
param(2) = dst
param(3) = 0.0d0
param(4) = winddens
param(5) = windvel
param(6) = 0.0d0
param(7) = 0.0d0
param(8) = 0.0d0
param(9) = 0.0d0
param(10) = 0.0d0
C
CALL UM520 (kouter, mdm%amjd, param,
: label, kout, ifail)
IF( ifail .LT. 0 )THEN
WRITE(kout,1000) 'UM520',ifail
STOP
ENDIF
C
CALL UA610 (katmo, mda, rzss, f107a, f107, max_kp,
: ap(1), ap(2), ap(3), ap(4), ap(5), ap(6),
: label, kout, ifail)
IF( ifail .LT. 0 )THEN
WRITE(kout,1000) 'UA610',ifail
STOP
ENDIF
C
C print the namelist
C
IF( list )then
WRITE(kout,1020)
WRITE(kout,unilib)
WRITE(kout,1020)
ENDIF
C
C format
C
1000 FORMAT(' *** Error in subroutine ',a5,', IFAIL =',i7,' ***')
1010 FORMAT(' *** Error in the namelist, IOSTAT =',i7,' ***')
1020 FORMAT(25('-'))
C
END
Results¶
SPENVIS
UNILIB Library
Version 2.22_YCH_initPrep
Generated by SPENVIS team
--- Main control parameters ---
UC160 (general constants):
Geoid major axis = 6378.16 km
Geoid minor axis = 6356.77 km
Mean Earth radius (Re) = 6371.20 km
Ecliptic inclination = 23.441512 deg
McIlwain Earth dipole = 0.311653 G/Re^3
UC190 (field line tracing):
Maximum step size = 477.8 km at one Re
Maximum "last" step size = 15.0 km
Minimum step size = 2.0 km
Altitude precision = 200. m
Magnetic field precision = 0.0006 %
UC192 (magnetic field evaluation):
Max. geomagnetic latitude = 85.0 deg
Minimum radius = 0.157 Re
(drift shell tracing):
Precision on L = 0.10000 %
Precision on the longitude = 0.05 deg
--- Geomagnetic field model ---
Model ( 0): DGRF 1995 Epoch 1995.
Order + 1 = 11
Calculation epoch = 1995.0 year
Colatitude of the dipole pole = 10.68 deg
Longitude of the dipole pole = -71.42 deg
Earth dipole moment = 0.302151 G/Re^3
Correction for the SAA drift = 0.00 deg
--- External magnetic field model ---
Model ( 0): None
Planetary activity index Kp = 0.0 (magnetopause check)
Date = 01-Jan-1995
Universal Time = 0.00 hrs
Greenwich angle of equinox = 100.18 deg
GEO direction of the Sun, X = -0.9200
Y = -0.0129
Z = -0.3917
Dipole tilt angle = -25.91 deg
--- Atmospheric model ---
Model (1): MSISE-90 neutral atmosphere
Calculation epoch = 1995.0 year
Universal Time = 0.00 deg
Sun spots number = 80.
Daily radio flux F10.7 (day-1) = 70.0 10-22 W m-2 Hz
81-Day avrg. radio flux F10.7 = 50.0 10-22 W m-2 Hz
Max. value of the 3-hour Kp = 3.0
Different Ap indices = 20. 20. 20. 20. 20. 20. 20.
Neutral species = H He Ar N2 O2 O. N.
Ionized species =
-------------------------
&UNILIB
KINNER = 0,
BLTIME = 1995.00000000000 ,
KOUTER = 0,
DATEMAGN = 1995, 2*1, 3*0,
VAL_KP = 0.000000000000000E+000,
DST = -30.0000000000000 ,
WINDDENS = 25.0000000000000 ,
WINDVEL = 300.000000000000 ,
KMFLG = 0,
KATMO = 1,
DATEATMO = 1995, 2*1, 3*0,
RZSS = 80.0000000000000 ,
F107 = 70.0000000000000 ,
F107A = 50.0000000000000 ,
MAX_KP = 3.00000000000000 ,
AP = 6*20.0000000000000 ,
KFLAG = 24*1, 2*0, 12*-1, 1, 11*0
/
-------------------------
UNILIB/tags/v3.02